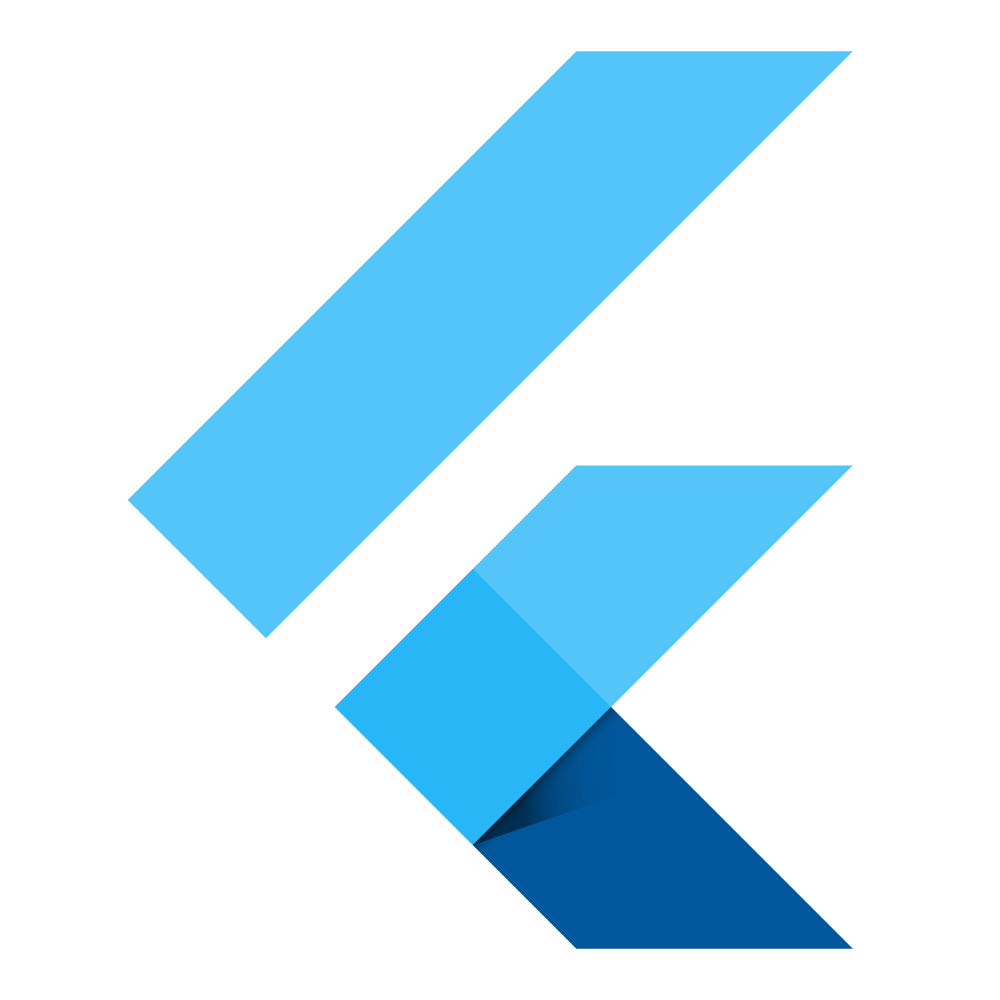
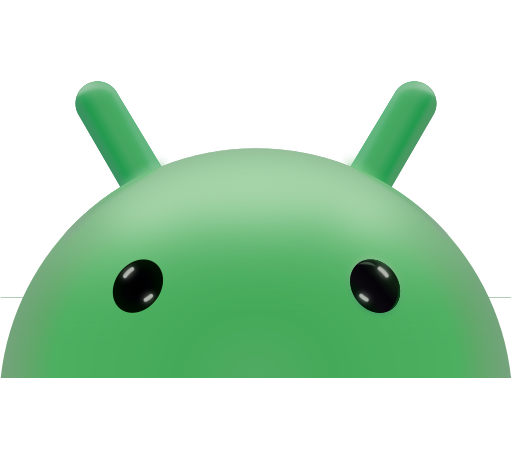
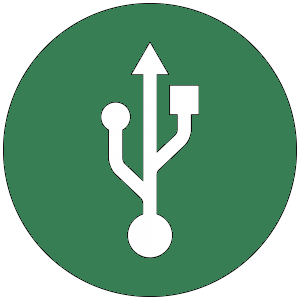
We recently integrated QR reader via USB. Our solution involved establishing a seamless connection, allowing the application to efficiently listen to the input stream through the USB port of Android Device. This implementation not only met the client’s requirements but also ensured a smooth and reliable interaction between the QR reader and the application.
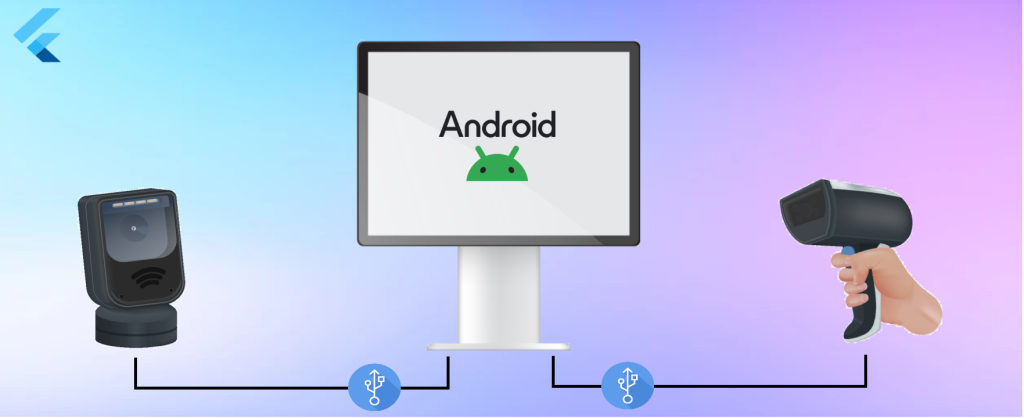
Firstly, we discovered that the scanner provides an option to connect to the Android device using either USB Keyboard or USB COM.
USB Keyboard
When a Barcode Scanner acts as a USB Keyboard input, it pretends to be a regular computer keyboard. Instead of using a dedicated connection, it connects to the computer through a USB port. When you scan a barcode, the scanner translates the information into keyboard strokes, as if someone typed it. This makes it easy to use because you don’t need special software—just plug it in like a keyboard, and the scanned data appears wherever you have your cursor, simplifying the process and making it compatible with different devices without extra fuss.
USB COM
A USB COM Port connection refers to the establishment of a communication link between a computer and a peripheral device, such as a barcode scanner or serial device, using the USB (Universal Serial Bus) interface. COM, short for Communication, denotes a serial communication port traditionally used in computing. The USB COM Port connection enables bidirectional data exchange, allowing the computer to send commands and receive data from the connected device. This standardized approach simplifies connectivity, providing a versatile and widely supported method for interfacing with various hardware components, enhancing interoperability and ease of use in diverse applications.
USB Keyboard vs USB COM
Choosing a USB COM port instead of a USB keyboard connection has its perks. Unlike a USB keyboard, which types directly into open text fields, the USB COM port lets us listen to the scanned data without automatically filling any text fields. This gives us more control. We can capture the scanned information and decide how to use it. It’s like being able to quietly listen to the scanner without it immediately talking to any text boxes. This way, we have the freedom to process the scanned results in a way that suits our specific needs without it automatically typing things wherever the cursor is.
Enabling USB COM Connectivity in Flutter
To achieve USB COM in Flutter, we found dependency usb_serial
that allows to interact with USB serial devices (FTDI or CDC).
To use this package first add following dependency to your pubspec.yaml
dependencies:
usb_serial: ^latest_version
Connect your Scanner device to your Android device using a USB port. To obtain a list of attached USB devices,
List<UsbDevice> usbDevices = await UsbSerial.listDevices(); List<UsbDevice> usbDevices = await UsbSerial.listDevices();
The package also includes a stream for listening to USB attach and detach events on the device.
UsbSerial.usbEventStream.listen((event){
//process USB event
});
After obtaining the list of USB devices, identify your Scanner device from the list. Subsequently, listen to the port to which the Scanner device is connected.
UsbDevice scannerDevice = usbDevices[index];
try{
UsbPort? port = await scannerDevice.create(); //return the UsbPort where scanner attached.
}catch (e){
//handle Error
}
After creating the UsbPort
, it’s essential to initially open the port to enable listening to incoming events from the Scanner. Once the UsbPort
is successfully opened, proceed to listen to the inputStream
of the UsbPort
.
bool? isOpen = await port?.open();
if(isOpen ?? false){
port?.inputStream?.listen((Uint8List event) async{
onUsbEvent(event); //process the incoming event here...
});
}
Handle the Scanner input,
void onUsbEvent(Uint8List event){
if(event.isEmpty) return;
String scanResult = utf8.decode(event);
//use this scanResult for further processing...
}
Conclusion
In summary, connecting a QR reader to a Flutter app via USB offers choices between USB Keyboard and USB COM. Opting for USB COM gives more control over handling scanned data. Using the usb_serial
package in Flutter makes connecting and communicating with USB devices straightforward, meeting various application requirements smoothly.